DNS(DOMAIN NAME SYSTEM)
DNS abbreviated as Domain Name System . Primary goal
of DNS is to make it easy for user to remember
simply the domain names of the
websites rather than the IP addresses of every site user wanted to visit.
Without DNS it’s very difficult for user to remember IP address of every site.
For example a user want to
visit a site, it will harder to remember 17.254.3.183
instead of just domain name of the site
aaple.com.
Domain Name System used because Web sites are essentially positioned
by their IP addresses. For
instance, when a user want to access a site,
computer sends a request for that site to adjacent DNS server,
which find the
IP address for that site. Then user’s
computer will connect to the server with that IP number.
 |
DNS Working |
DNS Architecture:
In early
days of internet when It was a small network, host names of the computers were
achieved through a
single server. As the number hosts increases load also
increased. At that time new system was needed
which reduce load on server also
allow many data types. Domain Name Service presented in 1984 became
this new
system. Using DNS, to reduce the load on any one server, all the host names
stored in database
which can be scattered between the multiple servers. The
potential size of DNS database is unlimited
because its database is scattered
also not decrease performance when multiple computers approach it.
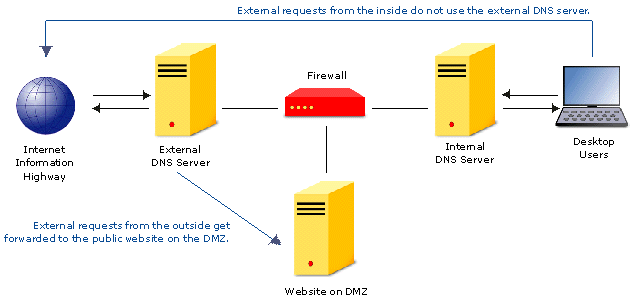 |
DNS Architecture |
Domain Namespace:
DNS namespace define a
hierarchical arrangement of domains that are combined to make a complete
domain name.
For instance, sub.secondary.com is a domain name where "com" is top-level
domain,
"secondary" is secondary domain name, and "sub" is
a sub-domain. The names make a hierarchical tree in
DNS database to form a
complete domain name is called Domain namespace
Many
organizations use their private domain namespace to create their own networks
which are not visible
on internet.
Recursive
query:Iterative query/ non recursive query:
In
recursive query , DNS server receive the user’s query then perform all the jobs
to achieve the answer
and give it back to the user. In this process, the DNS
server can also query the other DNS server's in the
internet on user’s behalf,
for the answer.If DNS server does not have the information that is required it
will
query to other servers until it get the required information or until
query fails.
Iterative query/ non recursive query:
In an iterative query, the DNS server
gives the best answer which based on cache or zone data. If the DNS
server does
not have the required information of user then it will
return a referral. DNS client send query to
the referral DNS server until it
met to a DNS server which will be authoritative for that query or until an
error condition. All DNS servers must follow non recursive query.
DNS(DOMAIN NAME SYSTEM)
DNS abbreviated as Domain Name System . Primary goal
of DNS is to make it easy for user to remember
simply the domain names of the
websites rather than the IP addresses of every site user wanted to visit.
Without DNS it’s very difficult for user to remember IP address of every site.
For example a user want to
visit a site, it will harder to remember 17.254.3.183
instead of just domain name of the site
aaple.com.
Domain Name System used because Web sites are essentially positioned
by their IP addresses. For
instance, when a user want to access a site,
computer sends a request for that site to adjacent DNS server,
which find the
IP address for that site. Then user’s
computer will connect to the server with that IP number.
 |
DNS Working |
DNS Architecture:
In early
days of internet when It was a small network, host names of the computers were
achieved through a
single server. As the number hosts increases load also
increased. At that time new system was needed
which reduce load on server also
allow many data types. Domain Name Service presented in 1984 became
this new
system. Using DNS, to reduce the load on any one server, all the host names
stored in database
which can be scattered between the multiple servers. The
potential size of DNS database is unlimited
because its database is scattered
also not decrease performance when multiple computers approach it.
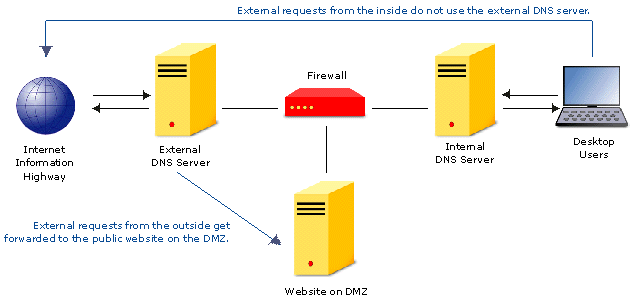 |
DNS Architecture |
Domain Namespace:
DNS namespace define a
hierarchical arrangement of domains that are combined to make a complete
domain name.
For instance, sub.secondary.com is a domain name where "com" is top-level
domain,
"secondary" is secondary domain name, and "sub" is
a sub-domain. The names make a hierarchical tree in
DNS database to form a
complete domain name is called Domain namespace
Many
organizations use their private domain namespace to create their own networks
which are not visible
on internet.
Recursive
query:Iterative query/ non recursive query:
In
recursive query , DNS server receive the user’s query then perform all the jobs
to achieve the answer
and give it back to the user. In this process, the DNS
server can also query the other DNS server's in the
internet on user’s behalf,
for the answer.If DNS server does not have the information that is required it
will
query to other servers until it get the required information or until
query fails.
Iterative query/ non recursive query:
In an iterative query, the DNS server
gives the best answer which based on cache or zone data. If the DNS
server does
not have the required information of user then it will
return a referral. DNS client send query to
the referral DNS server until it
met to a DNS server which will be authoritative for that query or until an
error condition. All DNS servers must follow non recursive query.
Posted at 08:47 |  by
viralnom